流式编程案例:使用Python进行数据处理
流式编程是一种编程范式,它将程序分解为一系列可组合的处理单元,每个处理单元负责一个特定的任务,并将输出传递给下一个处理单元。下面我们将以使用Python进行数据处理为例,展示流式编程的应用。
假设我们有一个包含大量文本数据的文件,我们需要对这些数据进行清洗、分词统计并生成词云图。
- 读取文件:从文本文件中读取数据。
- 数据清洗:去除特殊字符、空格等无关内容。
- 分词处理:对文本进行分词处理。
- 词频统计:统计词频。
- 生成词云图:根据词频生成词云图。
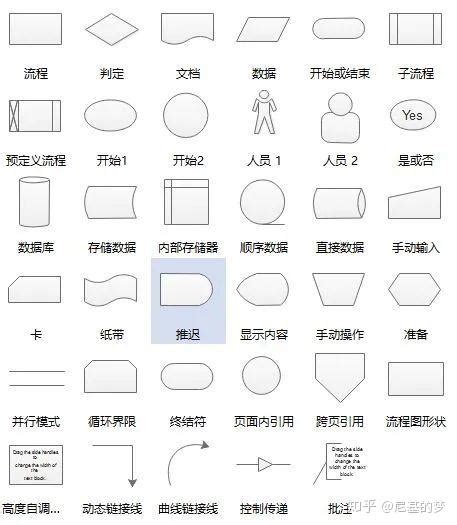
```python
import re
from collections import Counter
from wordcloud import WordCloud
import matplotlib.pyplot as plt
def read_file(filename):
with open(filename, 'r', encoding='utf8') as file:
return file.read()
def clean_text(text):
cleaned_text = re.sub(r'[^AZaz\s]', '', text)
cleaned_text = re.sub(r'\s ', ' ', cleaned_text)
return cleaned_text.lower()
def tokenize(text):
return text.split()
def count_words(tokens):
return Counter(tokens)
def generate_wordcloud(word_freq):
wordcloud = WordCloud(width=800, height=400, background_color='white').generate_from_frequencies(word_freq)
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
读取文件
data = read_file('sample.txt')
数据清洗
cleaned_data = clean_text(data)
分词处理
tokens = tokenize(cleaned_data)
词频统计
word_freq = count_words(tokens)
生成词云图
generate_wordcloud(word_freq)
```
通过上述流程设计和代码实现,我们可以方便地对文本数据进行处理,并生成词云图,从而直观展现关键词的分布情况。
流式编程能够使程序结构更清晰、易于维护,并且可以提高程序的灵活性和可扩展性。在实际项目中,我们可以根据具体需求设计流程,逐步完成复杂任务。建议在开发过程中充分利用流式编程思想,提高代码的可读性和可维护性。
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。